Deep Links
It's important to tell Superwall when a deep link has been opened. This enables a few things:
- Previewing paywalls on your device before going live.
- Deep linking to specific campaigns.
- Web Checkout Post-Checkout Redirecting
Setup
There are two ways to deep link into your app: URL Schemes and Universal Links (iOS only).
Adding a Custom URL Scheme
Open Xcode. In your info.plist, add a row called URL Types. Expand the automatically created Item 0, and inside the URL identifier value field, type your Bundle ID, e.g., com.superwall.Superwall-SwiftUI. Add another row to Item 0 called URL Schemes and set its Item 0 to a URL scheme you'd like to use for your app, e.g., exampleapp. Your structure should look like this:

With this example, the app will open in response to a deep link with the format exampleapp://. You can view Apple's documentation to learn more about custom URL schemes.
Adding a Universal Link (iOS only)
Only required for Web Checkout, otherwise you can skip this step.
Before configuring in your app, first create and configure your Stripe app on the Superwall Dashboard.
Add a new capability in Xcode
Select your target in Xcode, then select the Signing & Capabilities tab. Click on the + Capability button and select Associated Domains. This will add a new capability to your app.
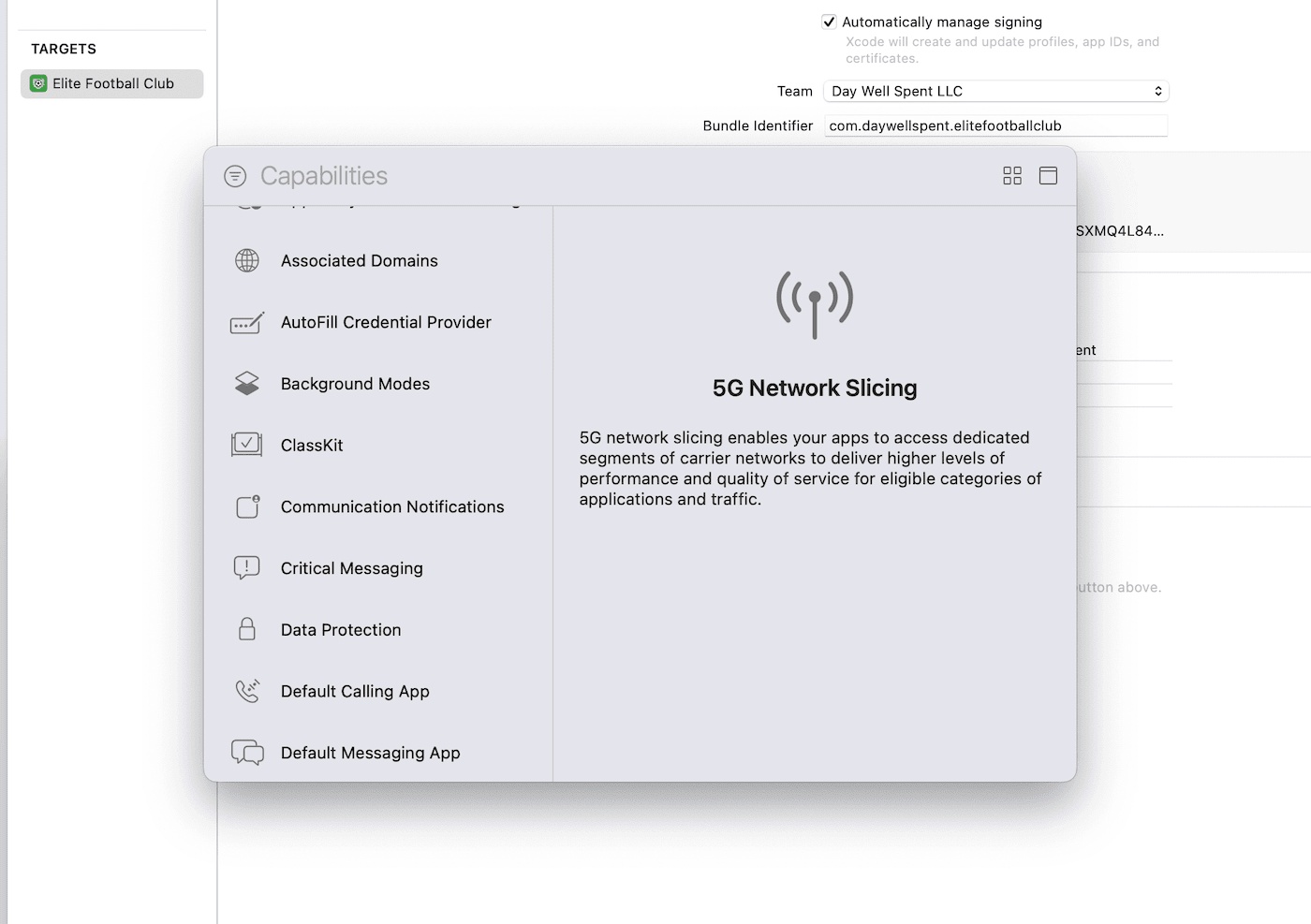
Set the domain
Next, enter in the domain using the format applinks:[your-web-checkout-url]
. This is the domain that Superwall will use to handle universal links. Your your-web-checkout-url
value should match what's under the "Web Paywall Domain" section.
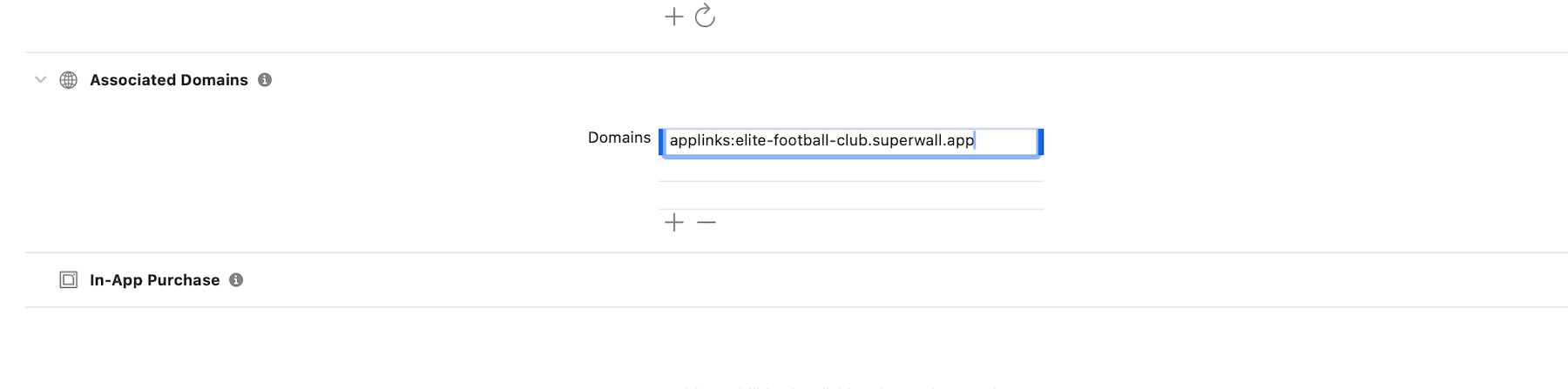
Testing
If your Stripe app's iOS Configuration is incomplete or incorrect, universal links will not work
You can verify that your universal links are working a few different ways. Keep in mind that it usually takes a few minutes for the associated domain file to propagate:
-
Use Branch's online validator: If you visit branch.io's online validator and enter in your web checkout URL, it'll run a similar check and provide the same output.
-
Test opening a universal link: If the validation passes from either of the two steps above, make sure visiting a universal link opens your app. Your link should be formatted as
https://[your web checkout link]/app-link/
— which is simply your web checkout link with/app-link/
at the end. This is easiest to test on device, since you have to tap an actual link instead of visiting one directly in Safari or another browser. In the iOS simulator, adding the link in the Reminders app works too:
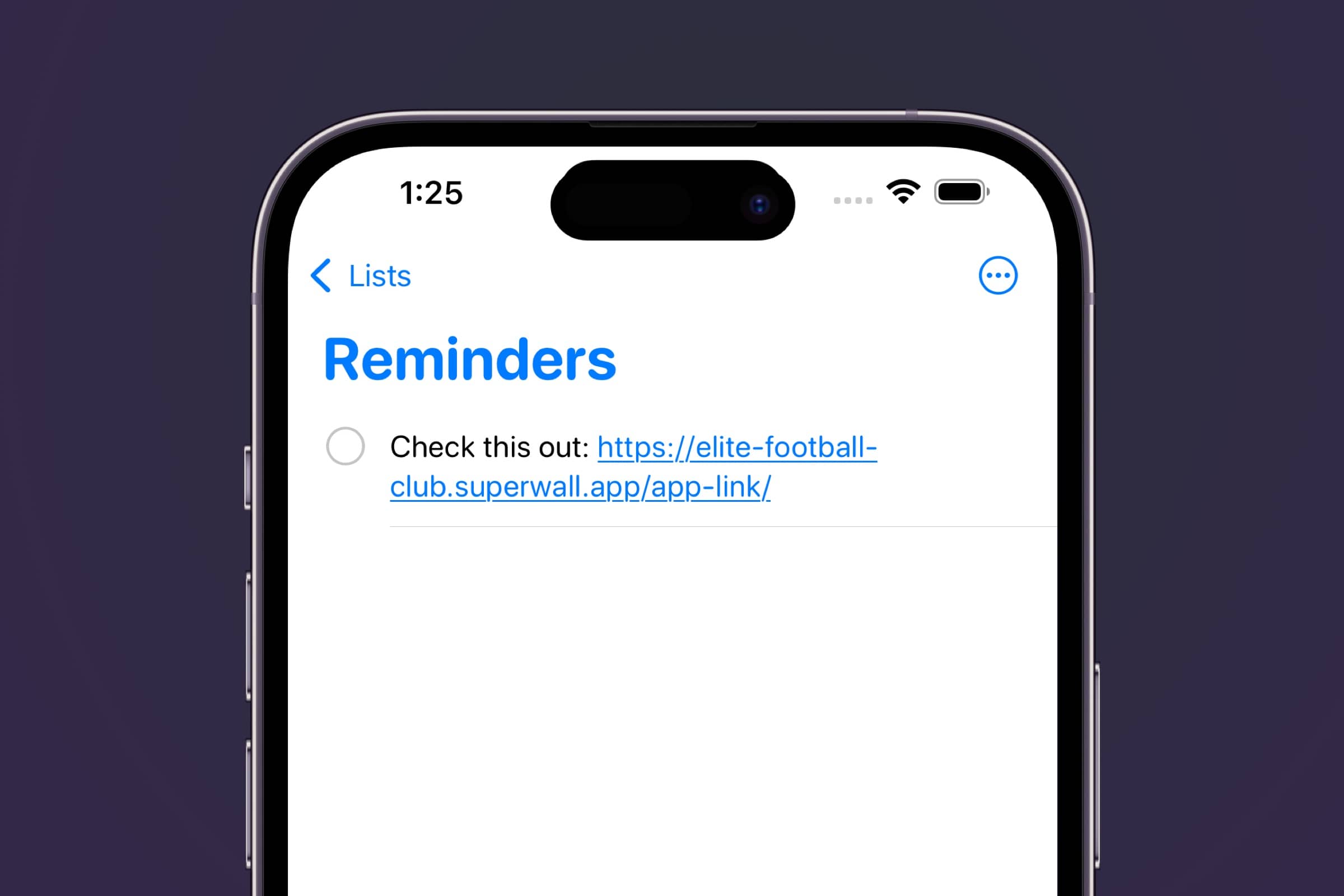
Handling Deep Links
Depending on whether your app uses a SceneDelegate, AppDelegate, or is written in SwiftUI, there are different ways to tell Superwall that a deep link has been opened.
Be sure to click the tab that corresponds to your architecture:
Previewing Paywalls
Next, build and run your app on your phone.
Then, head to the Superwall Dashboard. Click on Settings from the Dashboard panel on the left, then select General:
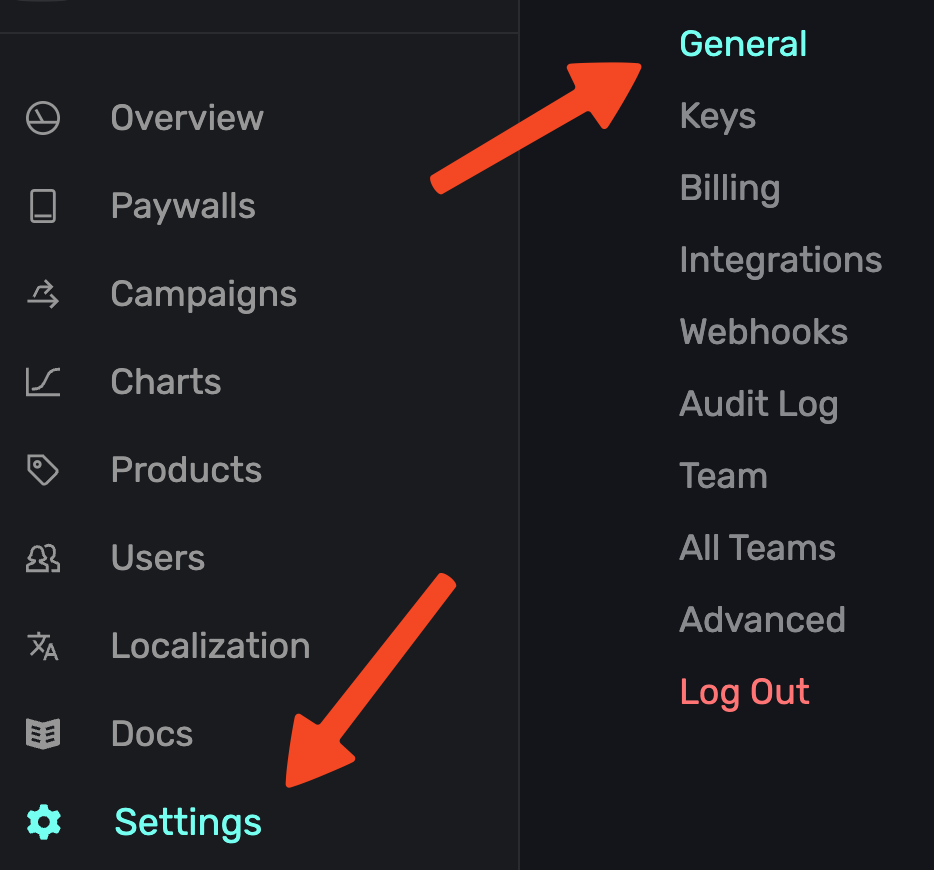
With the General tab selected, type your custom URL scheme, without slashes, into the Apple Custom URL Scheme field:
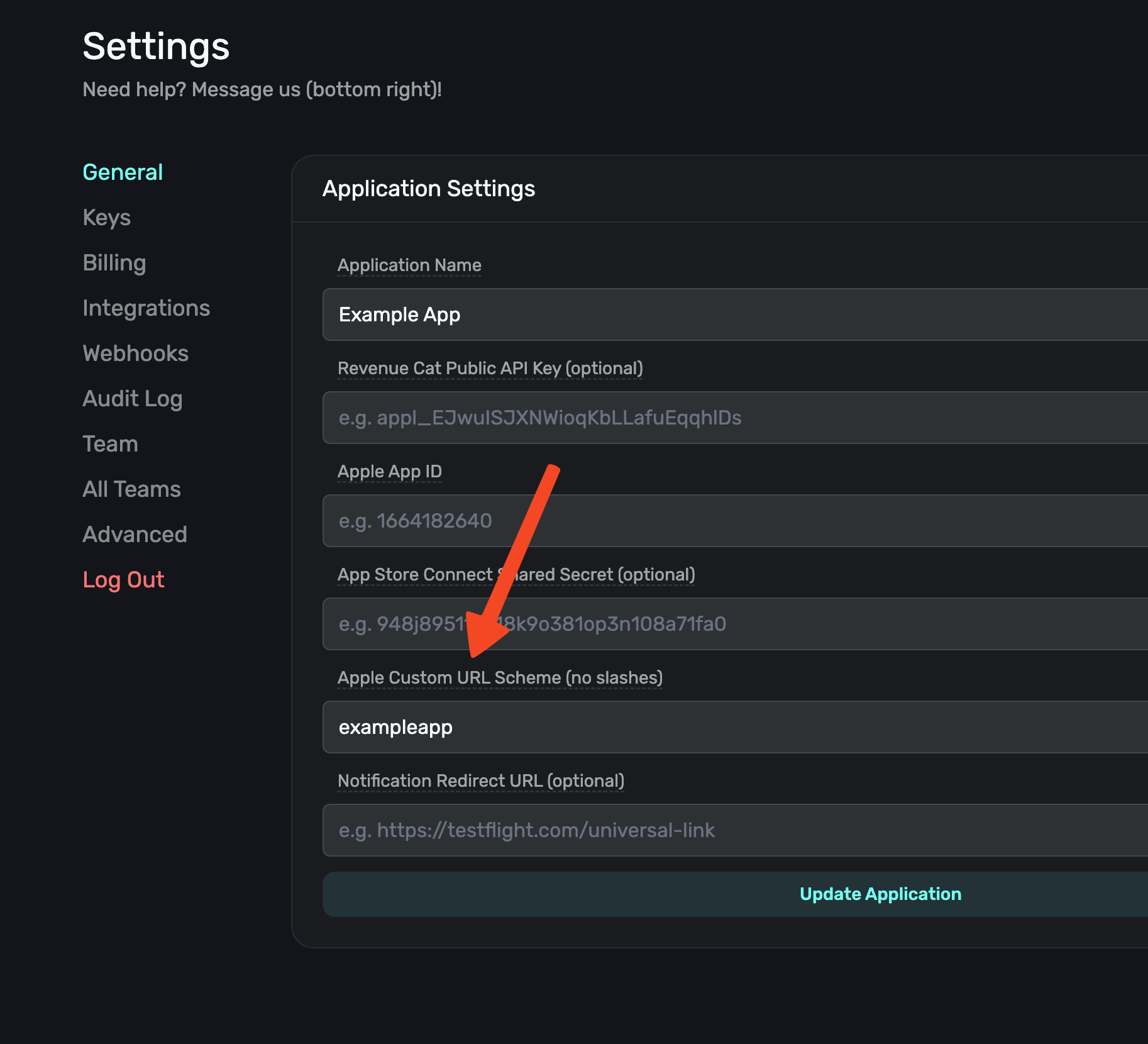
Next, open your paywall from the dashboard and click Preview. You'll see a QR code appear in a pop-up:
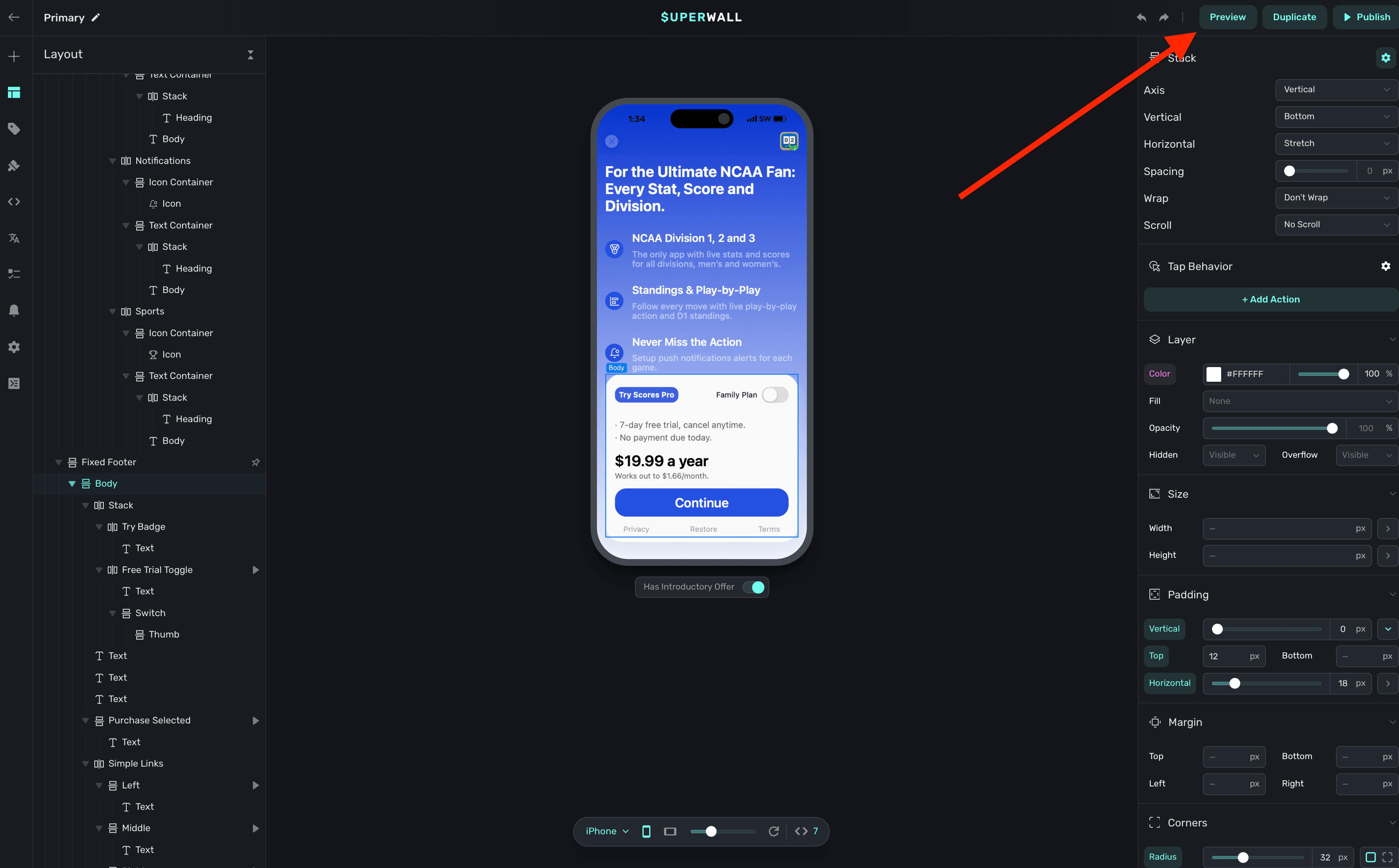
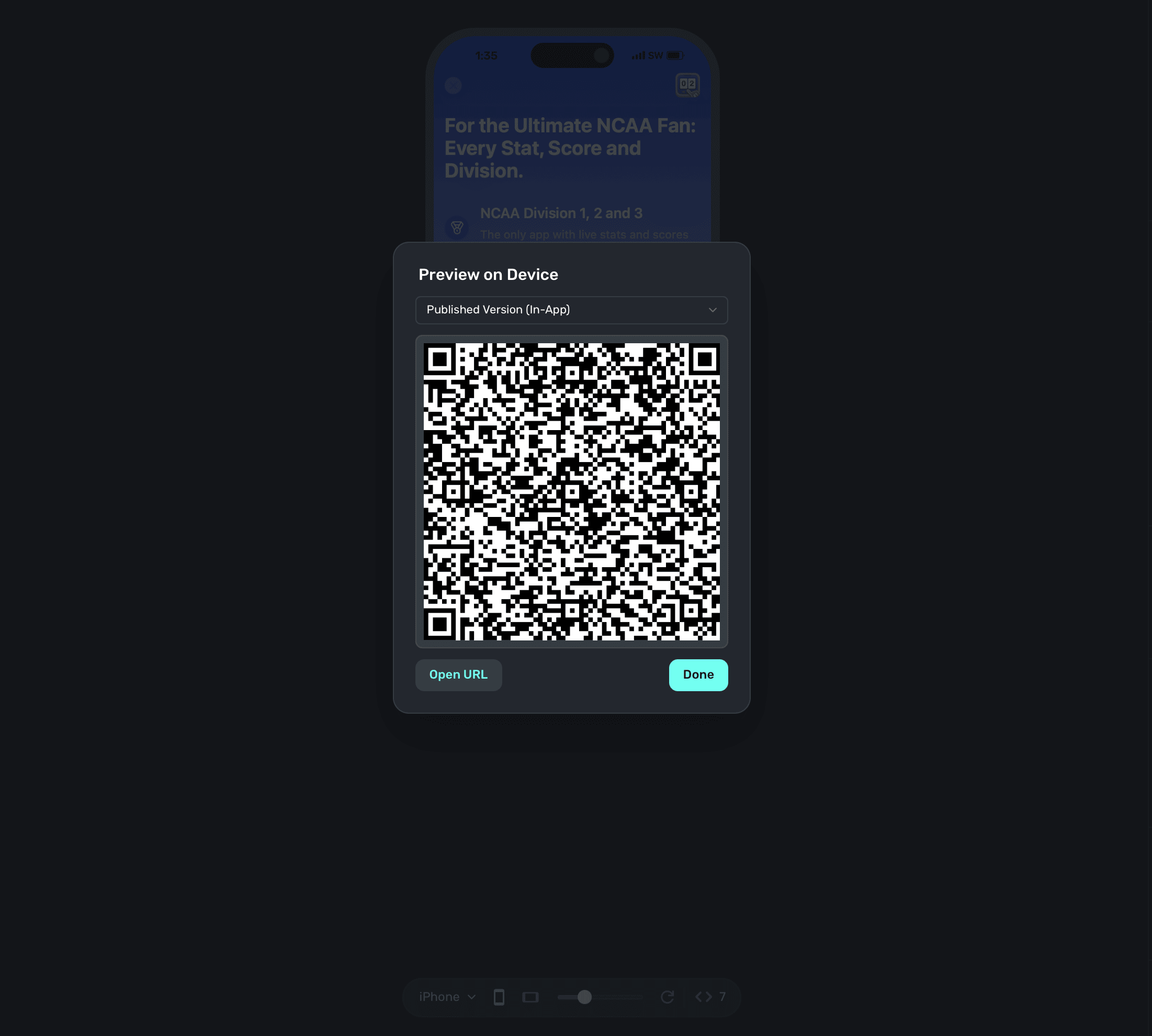
On your device, scan this QR code. You can do this via Apple's Camera app. This will take you to a paywall viewer within your app, where you can preview all your paywalls in different configurations.
Using Deep Links to Present Paywalls
Deep links can also be used as a placement in a campaign to present paywalls. Simply add deepLink_open
as an placement, and the URL parameters of the deep link can be used as parameters! You can also use custom placements for this purpose. Read this doc for examples of both.
How is this guide?
Custom Paywall Analytics
Learn how to log events from paywalls, such as a button tap or product change, to forward to your analytics service.
Custom Paywall Actions
You can set the click behavior of any element on a paywall to be a custom paywall action. This allows you to tie any tap in your paywall to hard-coded application logic.