Want to watch instead of read? Check this post out on YouTube.
Superwall's Flutter SDK is here! In this post, I'll show how to integrate Superwall in a Flutter app. Essentially, this is a three-step process. If you've installed Superwall in iOS or React Native projects, for example, you'll feel right at home.
The three primary steps are:
Installing Superwall's SDK.
Configuring the Superwall client inside a Flutter project.
And finally, presenting a paywall by registering a placement.
I've got a Flutter project to use here, and it only has one button. When we press it, Superwall will present a paywall:
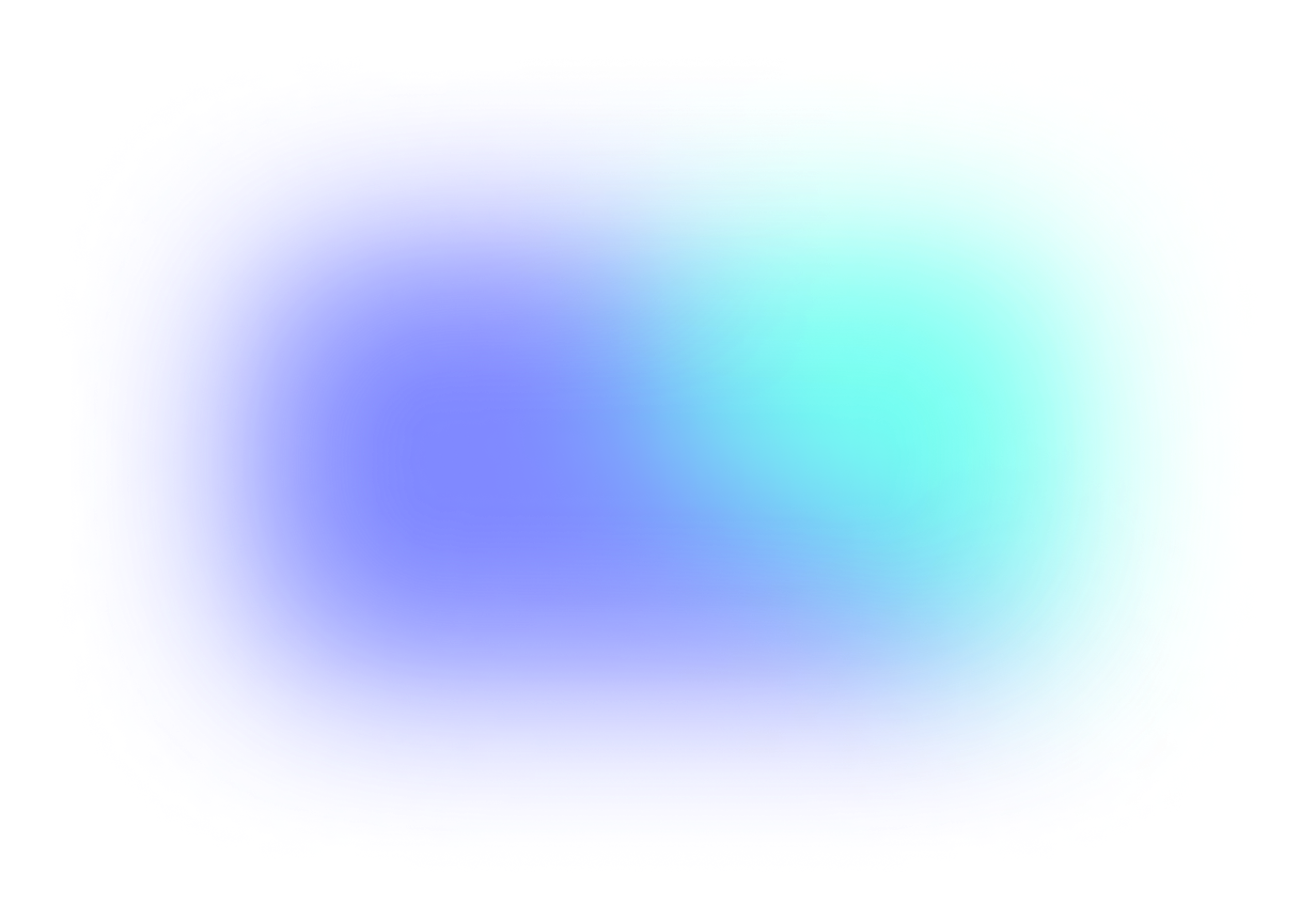
Before we get started, this post assumes you’ve already signed up for Superwall and added your products from App Store Connect or Google Play. If you need to do either of those, sign up for a free account here or learn how to add products to Superwall.
Finally, our minimum SDK support as of this writing is iOS 14, and SDK version 26 for Android.
Install the SDK
There are two ways you can install our Flutter SDK:
pubspec.yaml
First up, add superwallkit_flutter
as a dependency in your project's pubspec.yaml
file:
dependencies:
flutter:
sdk: flutter
# Add this:
superwallkit_flutter: ^2.0.0
Then, open terminal and fetch the package:
$ cd documents/myFlutterProjects
$ dart pub get
bash
Command line
You can also install our SDK straight from terminal:
$ cd documents/myFlutterProjects
$ flutter pub add superwallkit_flutter
bash
iOS deployment target
Superwall requires iOS 14 or above, so make sure you've set your iOS project's minimum deployment target accordingly. To do that, update its podfile
( MyFlutterProject -> ios -> podfile
) by adding platform
to the top, or updating it to iOS 14.0 or above if it's already there:
platform :ios, '14.0'
bash
If you haven't installed your iOS dependencies yet, you may also need to run pod install
too:
$ cd documents/myFlutterProjects/ios
$ pod install
bash
Be aware that, depending on your project setup, you may need to open up Xcode and set the same minimum deployment version there as well. It should match what you've set in your podfile
:
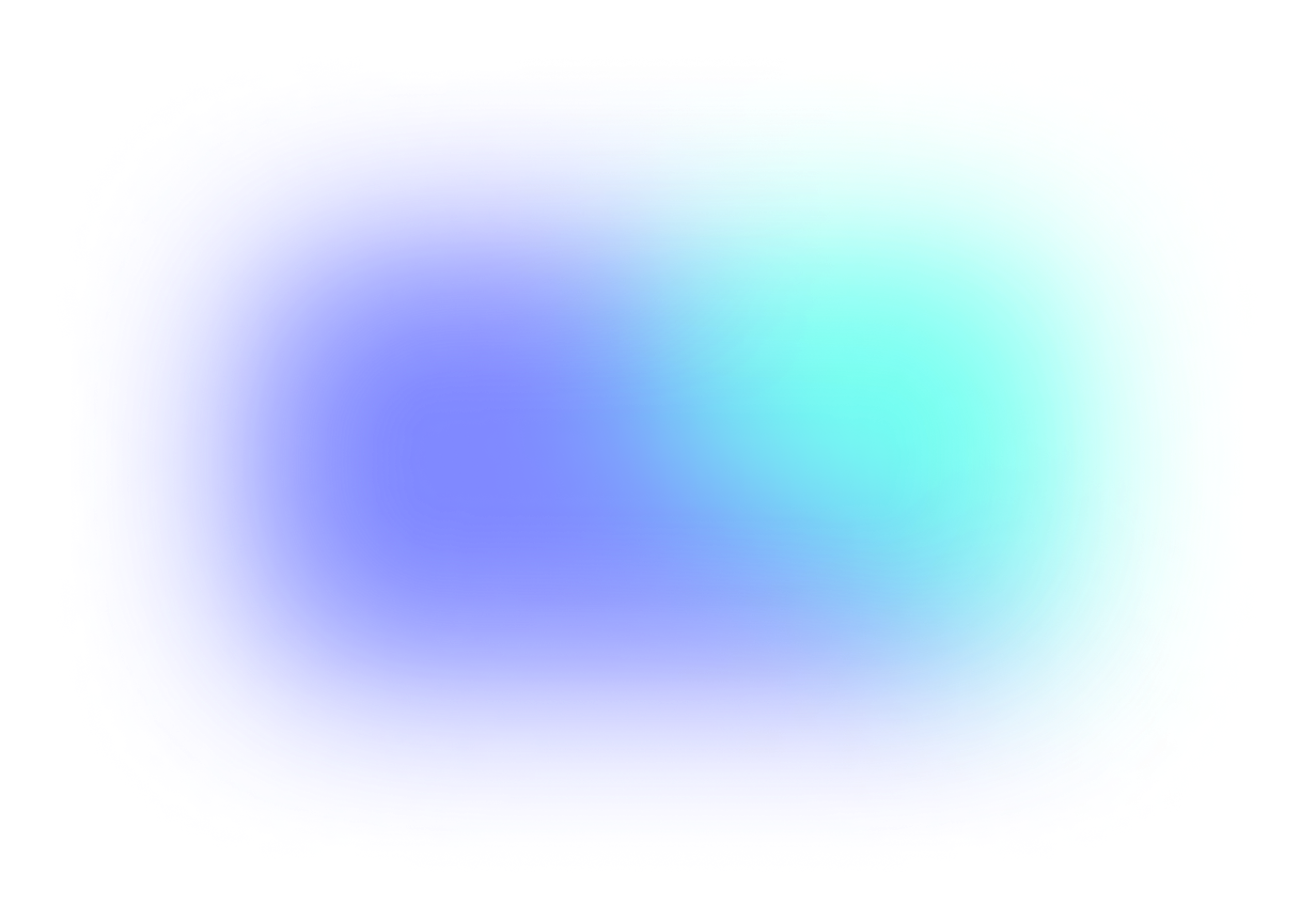
Android configuration
For Android, make sure your setup meets the following requirements:
Minimum SDK Version: 26 or higher
Compile SDK Target: 34
You can check, and update, SDK versions in your project's android/app/build.gradle
file:
android {
...
compileSdkVersion 34
...
defaultConfig {
...
minSdkVersion 26
...
}
}
gradle
To use SDK target 34, you'll need to make sure you're using the correct versions of Gradle and the Android Gradle Plugin:
Gradle Version: 8.6 or higher
Android Gradle Plugin Version: 8.4 or higher
Update your gradle/wrapper/gradle-wrapper.properties
file to use the latest Gradle version:
distributionUrl=https\://services.gradle.org/distributions/gradle-8.6-bin.zip
properties
Also, check that your android/build.gradle
file uses the latest Android Gradle plugin too:
plugins {
id 'com.android.application' version '8.4.1' apply false
}
gradle
Ensure project builds
Installation is all done now! It's a perfect time to go to the root of your project and make sure everything builds and runs just fine:
$ cd documents/myFlutterProjects
$ flutter run ios
bash
Configure Superwall
Now, open main.dart
(usually under lib -> main.dart
) and import Superwall at the top:
import 'package:superwallkit_flutter/superwallkit_flutter.dart';
dart
Call configure
on Superwall with your API key for the project. You'll have one for Android, and one for iOS:
String apiKey = Platform.isIOS ? "MY_IOS_API_KEY" : "MY_ANDROID_API_KEY";
Superwall.configure(apiKey);
dart
Here's a complete code sample:
import 'dart:io';
import 'package:superwallkit_flutter/superwallkit_flutter.dart';
class _MyAppState extends StatelessWidget {
@override
void initState() {
String apiKey = Platform.isIOS ? "MY_IOS_API_KEY" : "MY_ANDROID_API_KEY";
Superwall.configure(apiKey);
}
// Rest of app code
}
dart
If you don't know what your API keys are, head to Superwall's dashboard and choose either your iOS or Android app that was created for your Flutter project. Then go to Settings -> Keys -> Public API Key
to copy your app's key.
Present a paywall
Finally, we'll register a placement with Superwall to trigger a paywall. By default, every campaign comes with a placement we can use called campaign_trigger
. We'll use that and create a function to register it:
void registerDemoPlacement() {
Superwall.shared.registerPlacement('campaign_trigger', feature: () {
// access pro feature
});
}
dart
Now, when the button is tapped — we'll get a default paywall to show:
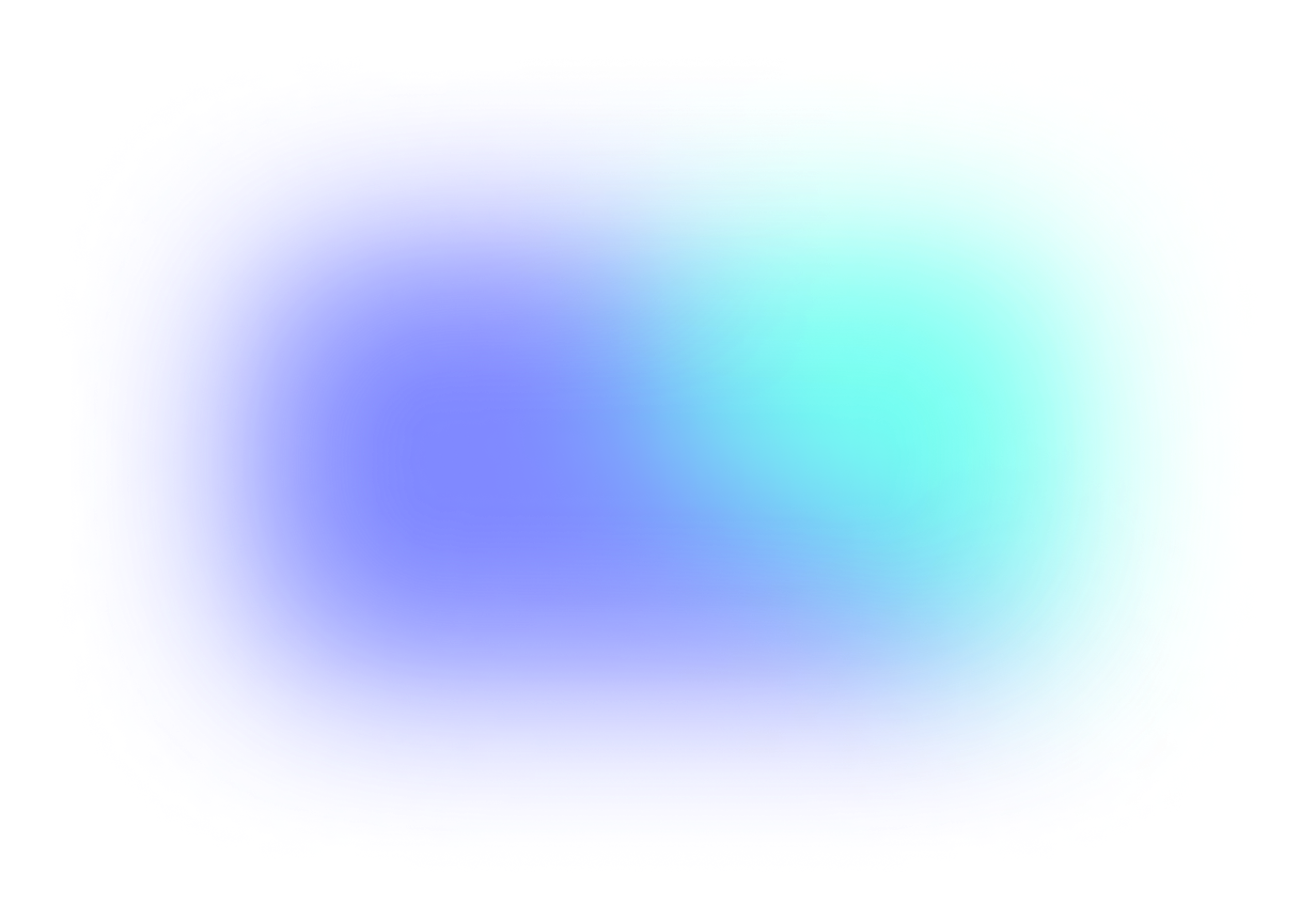
And that's it! Superwall is all set, and you're ready to start growing your revenue, begin running paywall experiments and more.
If it helps, here is the entire source code for the project's main.dart
file:
import 'package:flutter/material.dart';
import 'package:superwallkit_flutter/superwallkit_flutter.dart';
import 'dart:io';
void main() {
runApp(const MyApp());
}
class MyApp extends StatefulWidget {
const MyApp({super.key});
@override
_MyAppState createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> {
@override
void initState() {
super.initState();
String apiKey = Platform.isIOS ? "MY_IOS_API_KEY" : "MY_ANDROID_API_KEY";
Superwall.configure(apiKey);
}
void registerDemoPlacement() {
Superwall.shared.registerPlacement('campaign_trigger', feature: () {
// Access pro
});
}
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
ElevatedButton(
onPressed: registerDemoPlacement,
child: const Text('Present Paywall'),
),
],
),
),
),
);
}
}
dart
If you're new to Superwall, you may be wondering how all of this is working. What's a placement, and why do you register them? Read the next section for a quick explanation, or if you've used Superwall before — you're all done!
Understanding Placements
With Superwall, paywalls can be triggered by specific actions (i.e. a placement), built-in placements such as "first install", or even programmatically via our SDK.
Paywalls are usually displayed based on placements tied to a campaign. Each campaign can have one (or many) paywalls linked to it. Placements serve as markers for anything in the app that requires a subscription, or "pro" entitlements, to access, and that's often referred to as being "paywalled".
So, in a caffeine tracking app, placements might include:
When caffeine is logged,
caffeineLogged
.When selecting a custom icon,
customIconSelected
.And so on...
This setup provides a lot of flexibility since Superwall lets you remotely control feature access (and paywalls!) without needing to push app updates.
Want to make caffeine logging free for a weekend? Just pause the placement, or make the paywall "non-gated". You can set up complex filters, rules and more to decide when placements should be triggered - or even use unique paywalls per placement.
As I mentioned in the steps above, when you create an app in Superwall there will be an example campaign and placement to help you get started. Feel free to use it as a foundation, and remember, there's plenty more to explore — check out our documentation for further details or watch our Youtube Channel!
Finishing up
That's all it takes to get things running with Flutter. If you ran into any issues with your setup, don't hesitate to reach out through Superwall’s support channels or send me a message on Twitter. We're here to help and excited to watch you grow your revenue using our Flutter SDK!